The JsonStore is used to store data to permanent memory so it can be read later.
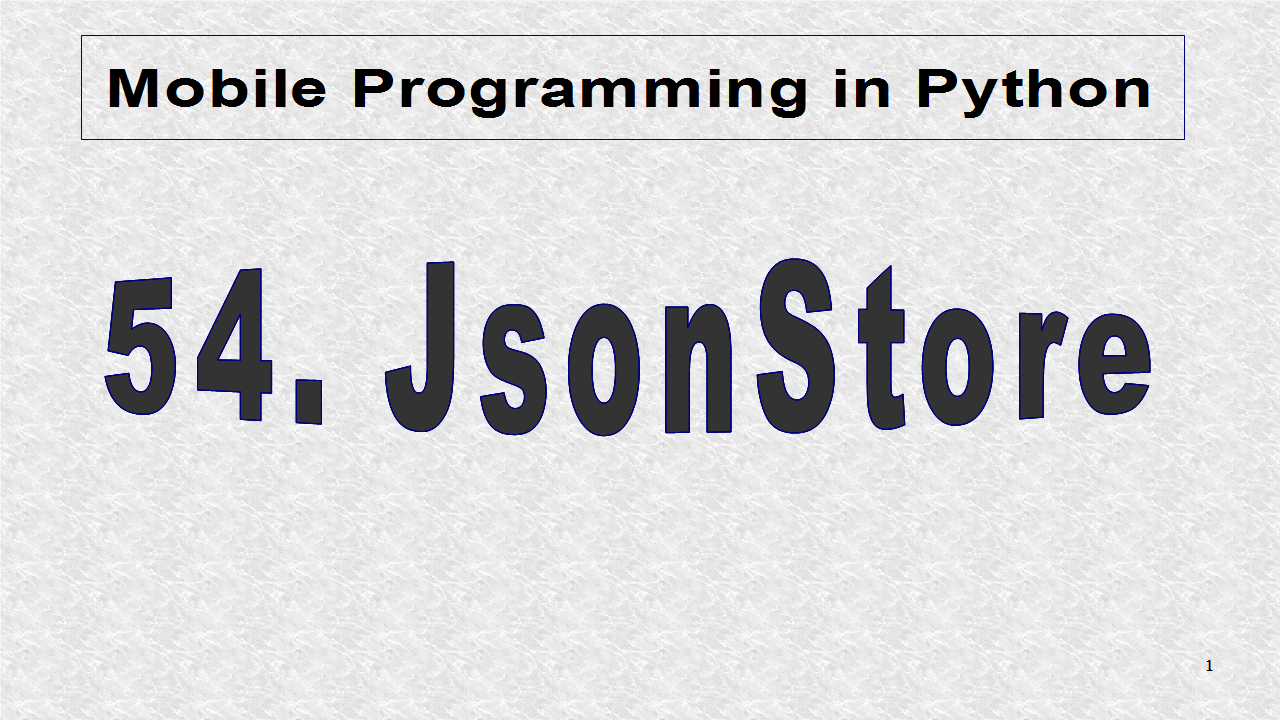
The imports include the App to create an app, and the GridLayout for the root. Each of the grids will be a Label. We need a few graphics classes. The Window is used to find the size of screen. Finally, JsonStore is needed for saving and reading of data, from the json file.
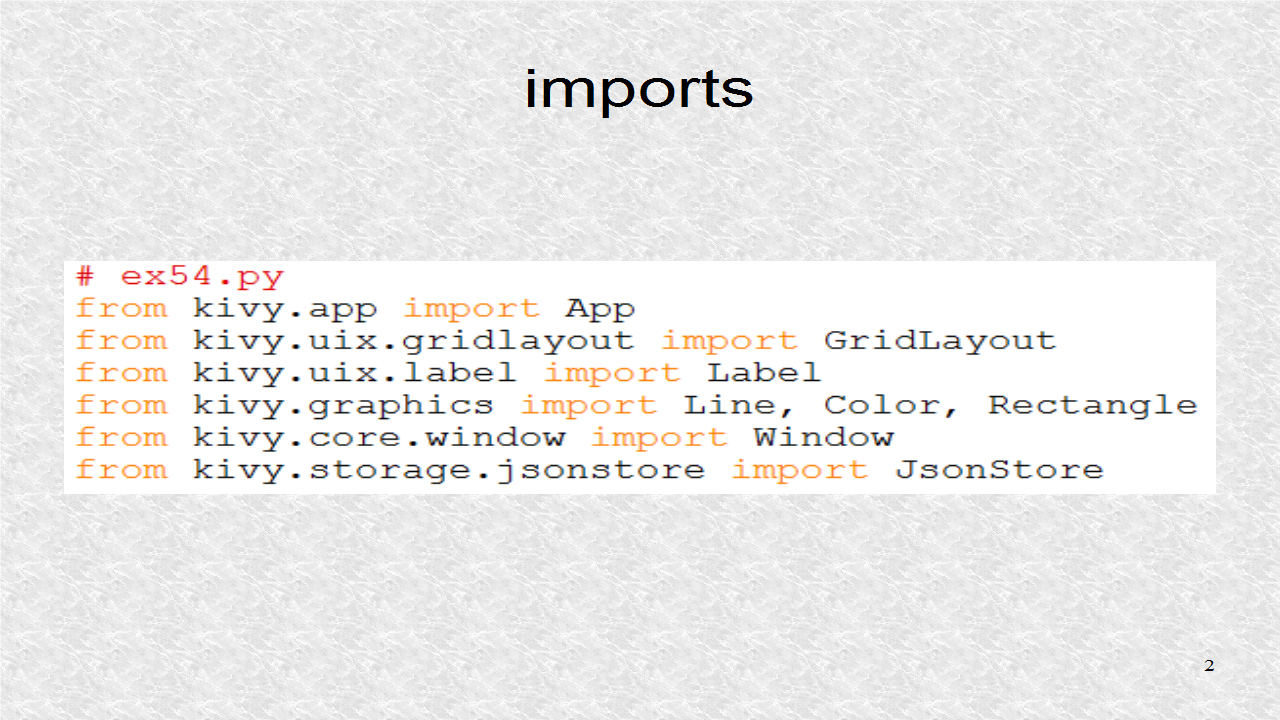
In the build() of the app, we create a store variable, pointing to the json file. Here, four dictionary elements are written to it.
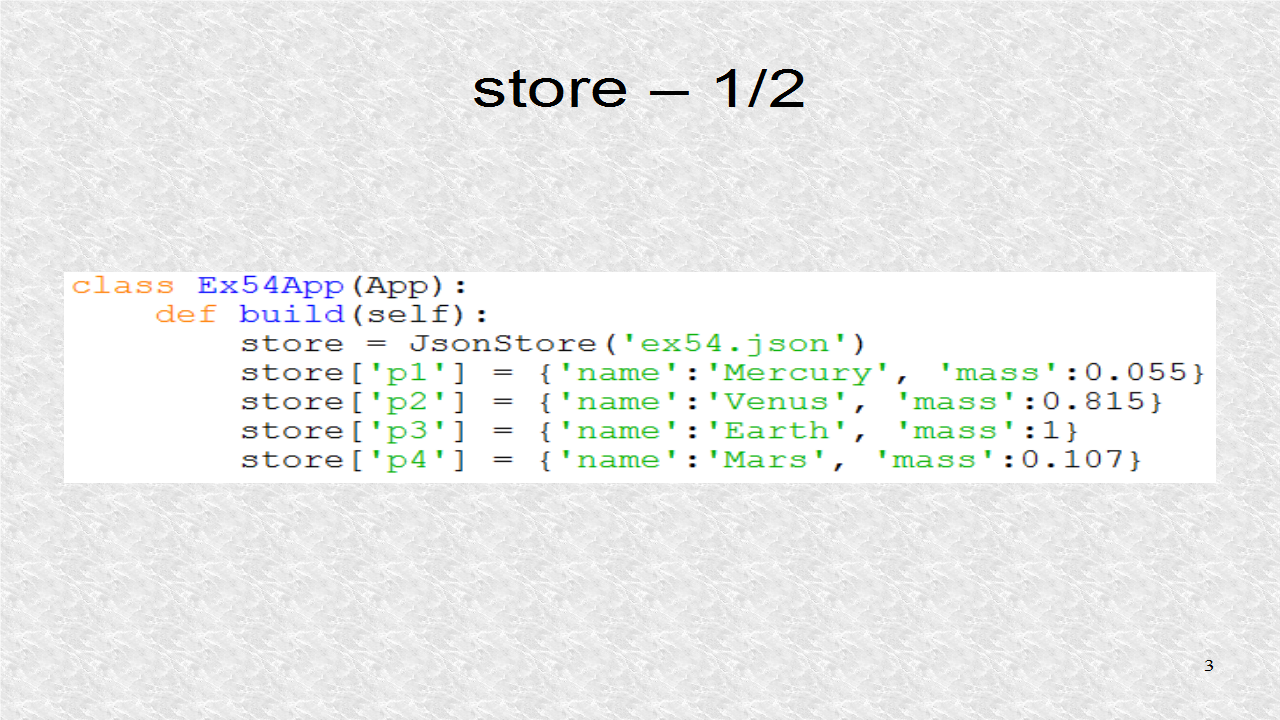
These are the last four elements which are saved to json file.
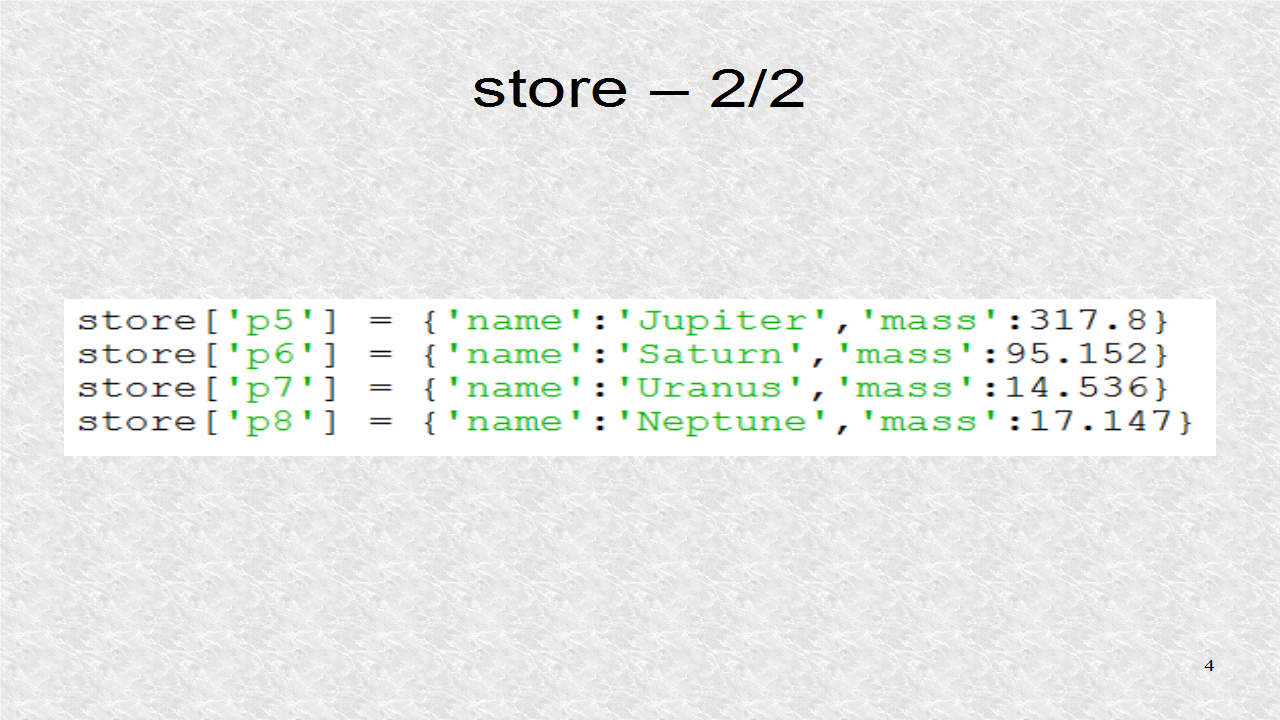
The variable y0, indicates the y-separation of horizontal lines. There will be 9 such lines separating the rows of the grid. The variable y, initially, points to top of the screen. It is moved down by y0 each time a new horizontal line is drawn. The root is a GridLayout with 2 columns. Str and Str1 are templates, for header and non-header labels.
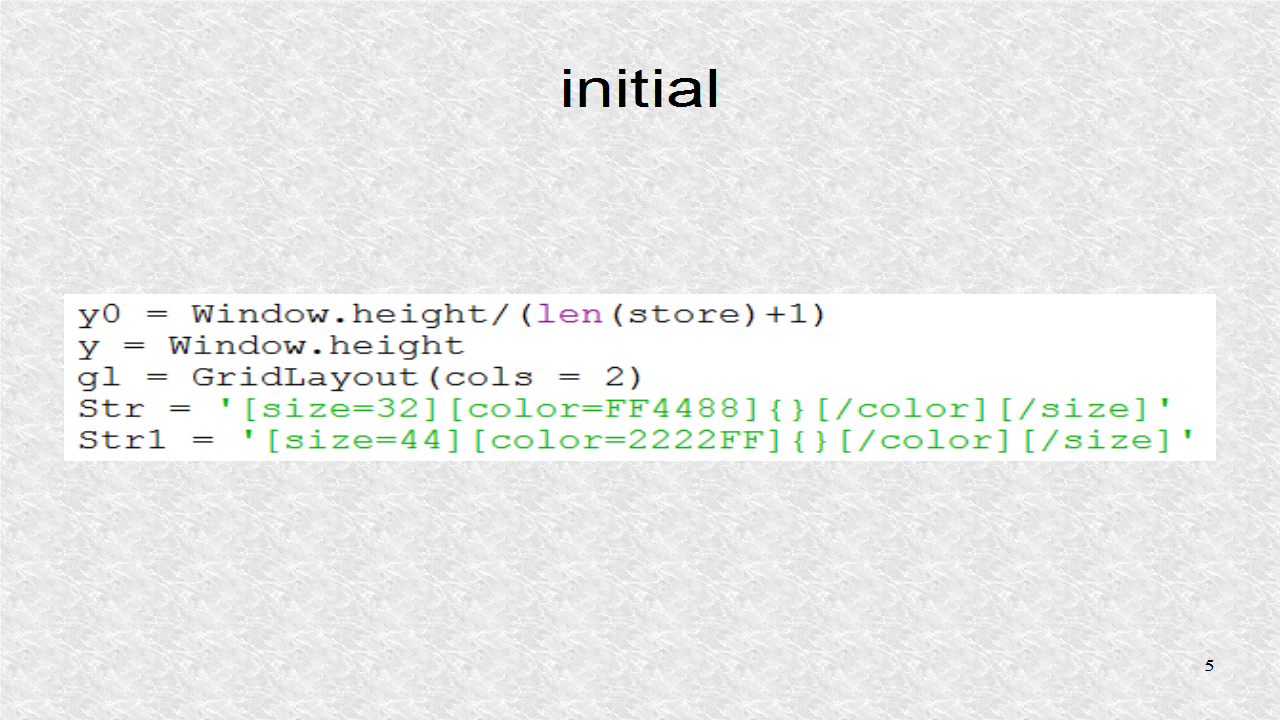
The background color is set. The left header is written.
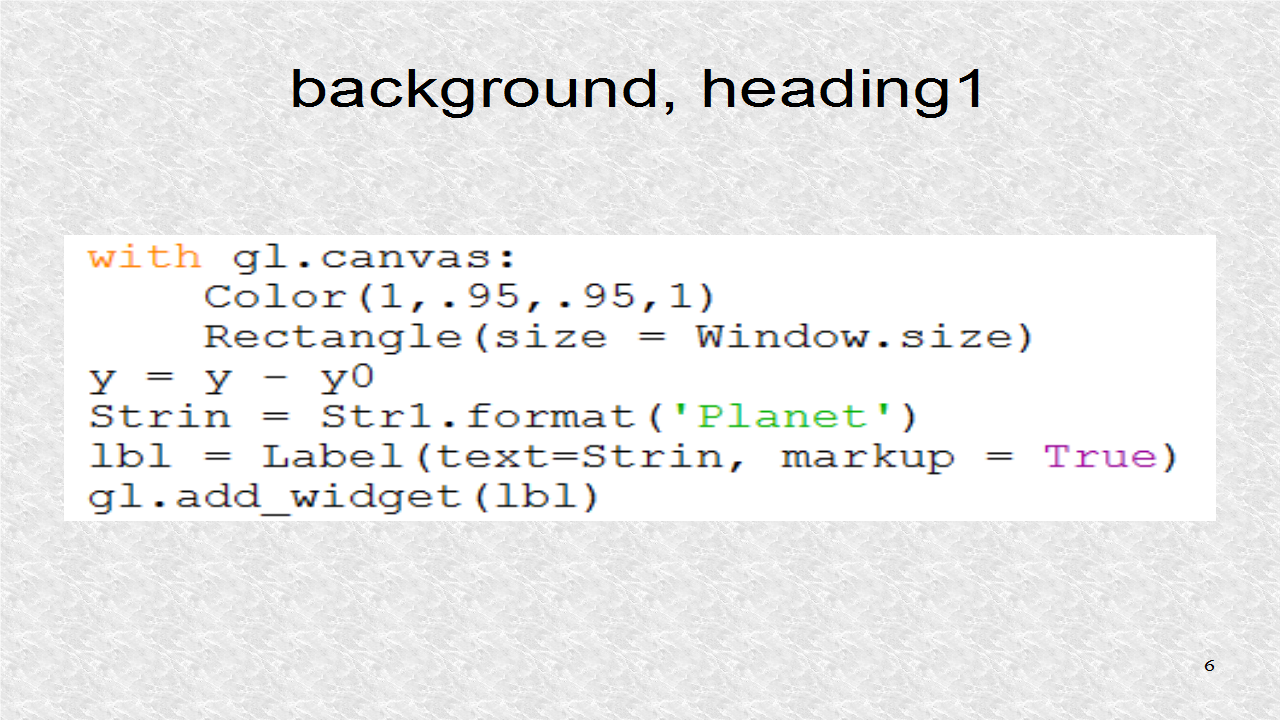
The right header is written, as well as a horizontal line, just below the header labels.
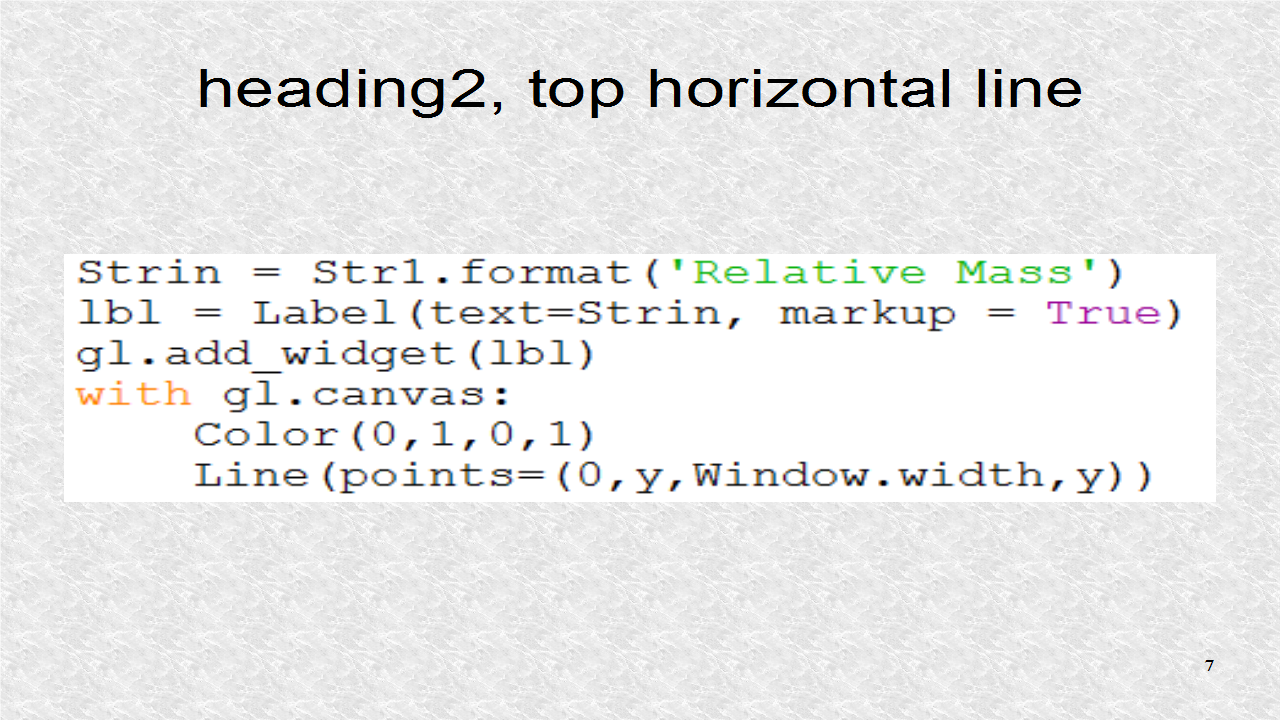
We iterate, over the 8 elements, of the store. Since store is a dictionary structure, that is, a dictionary of dictionaries, it is not sorted. That is why the sorted() function is used. The left-most label is written.
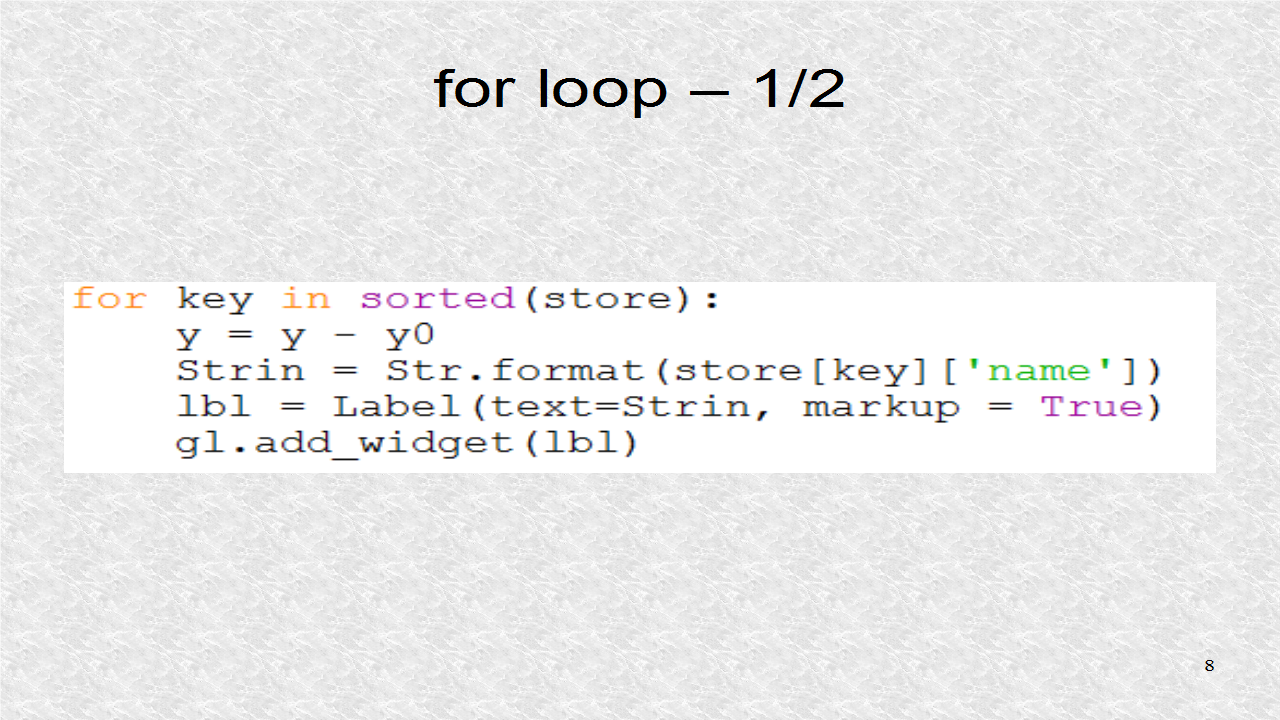
The right label is written, as well as the horizontal line just underneath acting as a divider. Besides this, we have the main code with the run() function.
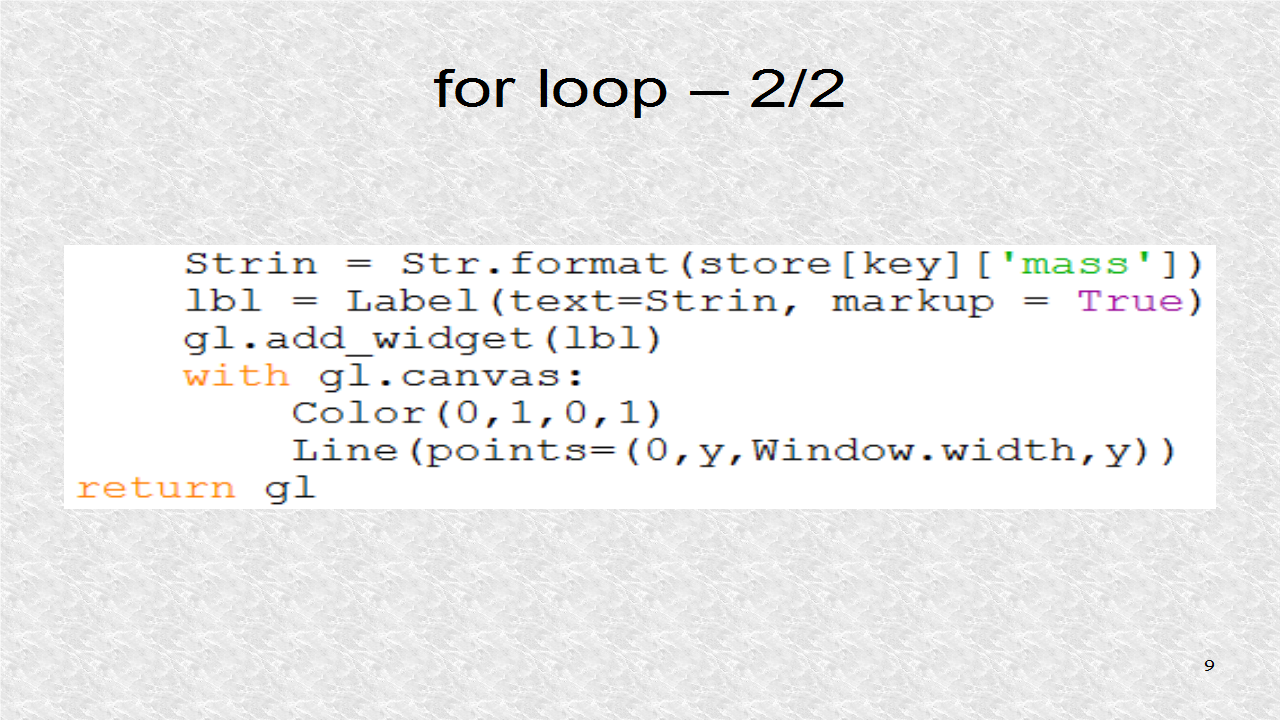
# ex54.py from kivy.app import App from kivy.uix.gridlayout import GridLayout from kivy.uix.label import Label from kivy.graphics import Line, Color, Rectangle from kivy.core.window import Window from kivy.storage.jsonstore import JsonStore class Ex54App(App): def build(self): store = JsonStore('ex54.json') store['p1'] = {'name':'Mercury', 'mass':0.055} store['p2'] = {'name':'Venus', 'mass':0.815} store['p3'] = {'name':'Earth', 'mass':1} store['p4'] = {'name':'Mars', 'mass':0.107} store['p5'] = {'name':'Jupiter','mass':317.8} store['p6'] = {'name':'Saturn','mass':95.152} store['p7'] = {'name':'Uranus','mass':14.536} store['p8'] = {'name':'Neptune','mass':17.147} y0 = Window.height/(len(store)+1) y = Window.height gl = GridLayout(cols = 2) Str = '[size=32][color=FF4488]{}[/color][/size]' Str1 = '[size=44][color=2222FF]{}[/color][/size]' with gl.canvas: Color(1,.95,.95,1) Rectangle(size = Window.size) y = y - y0 Strin = Str1.format('Planet') lbl = Label(text=Strin, markup = True) gl.add_widget(lbl) Strin = Str1.format('Relative Mass') lbl = Label(text=Strin, markup = True) gl.add_widget(lbl) with gl.canvas: Color(0,1,0,1) Line(points=(0,y,Window.width,y)) for key in sorted(store): y = y - y0 Strin = Str.format(store[key]['name']) lbl = Label(text=Strin, markup = True) gl.add_widget(lbl) Strin = Str.format(store[key]['mass']) lbl = Label(text=Strin, markup = True) gl.add_widget(lbl) with gl.canvas: Color(0,1,0,1) Line(points=(0,y,Window.width,y)) return gl if __name__=='__main__': Ex54App().run()
We can always open the json file which is saved to the hard drive on desktop. Here is the first four elements, with newlines manually added for clarity.
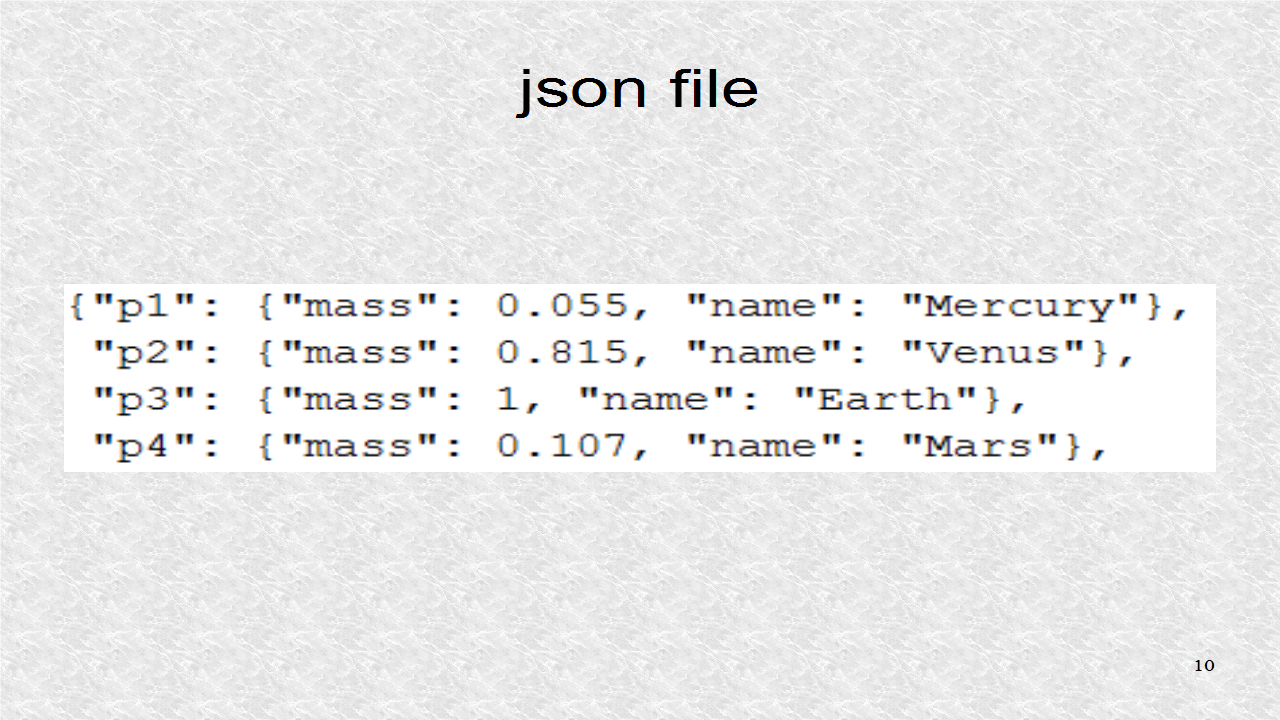
This result shows the header and the 8 rows with the data. The header row and data rows are different colors since they are based on different string templates, with different color and size markup. We can also see the horizontal lines dividing the rows.
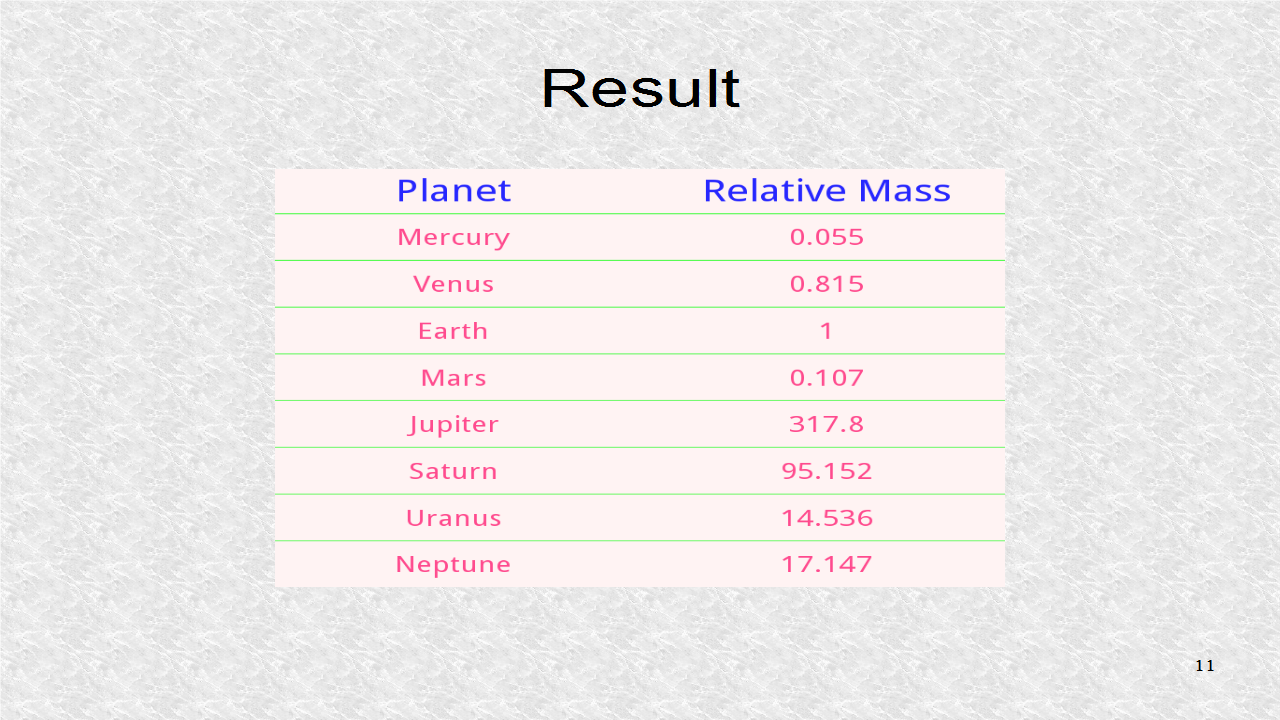
Good Post! Thank you so much for sharing this pretty post, it was so good to read and useful to improve my knowledge as updated one, keep blogging.
ReplyDeletePython Training in electronic city, Bangalore | #pythontraininginelectroniccity #pythontraining
DataSciencewithPythonTraininginelectroniccity,Bangalore | #datasciencewithpythontraininginelectroniccity #datasciencewithpythontraining
Good Post! Thank you so much for sharing this pretty post, it was so good to read and useful to improve my knowledge as updated one, keep blogging.
ReplyDeletePython Training in electronic city
thank you .
ReplyDeletevisit
web programming tutorial
welookups
nice information for beginners.thank you.
ReplyDeletejavacodegeeks
welookups python