We can use Kivy Logger to log messages. These messages will be displayed, on console, as well as being saved to a file.
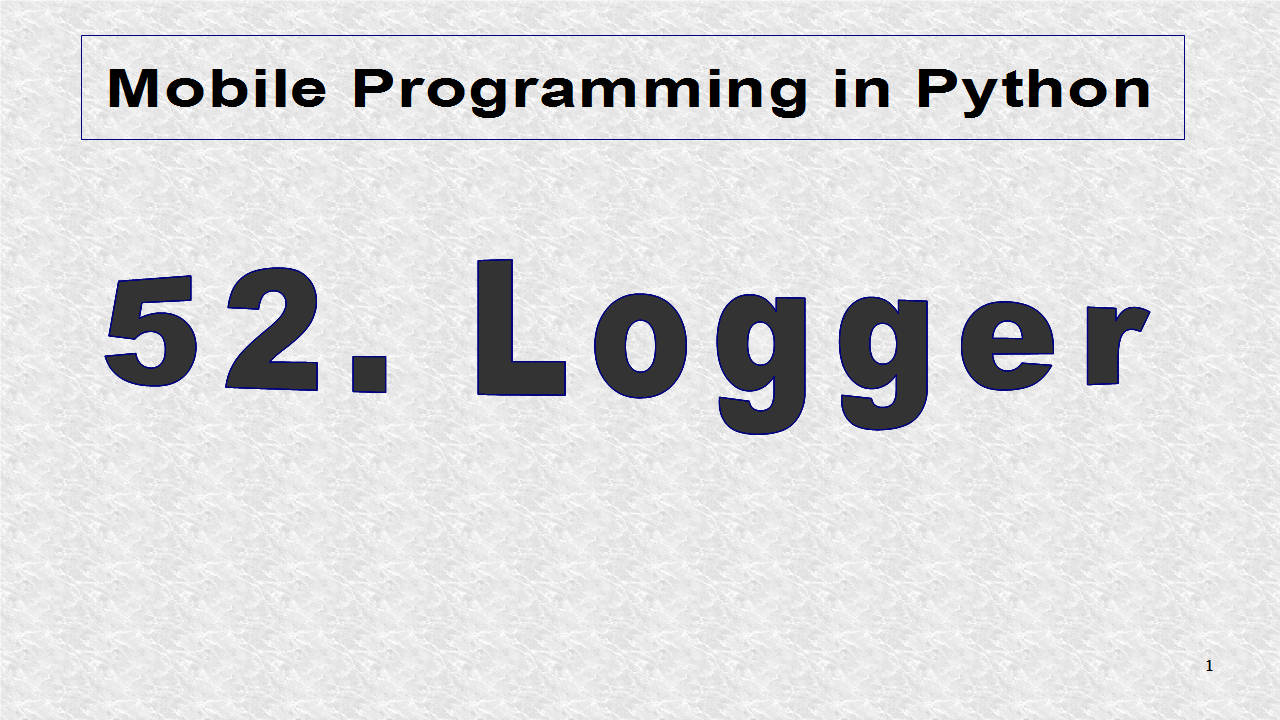
This example is based on example 27, on Widgets. The additional imports are for Logger and ctime. ctime is part of the standard python library time module.
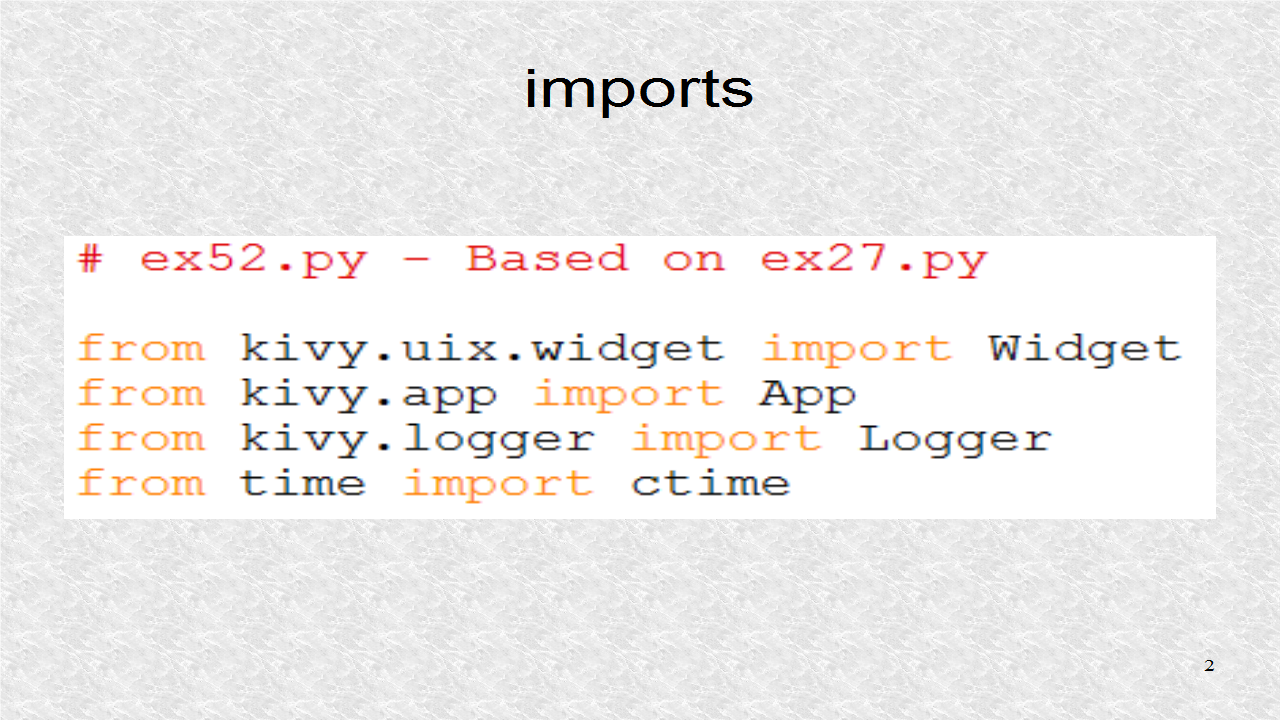
This is the first half of the log function which is used as a decorator. It first set's the counter to zero. This variable is attached to the particular function so there is such a variable for each function decorated. Whenever a decorated function is called, it will call decor() instead or whatever the name of the decorated function is. This function first increments the internal counter.
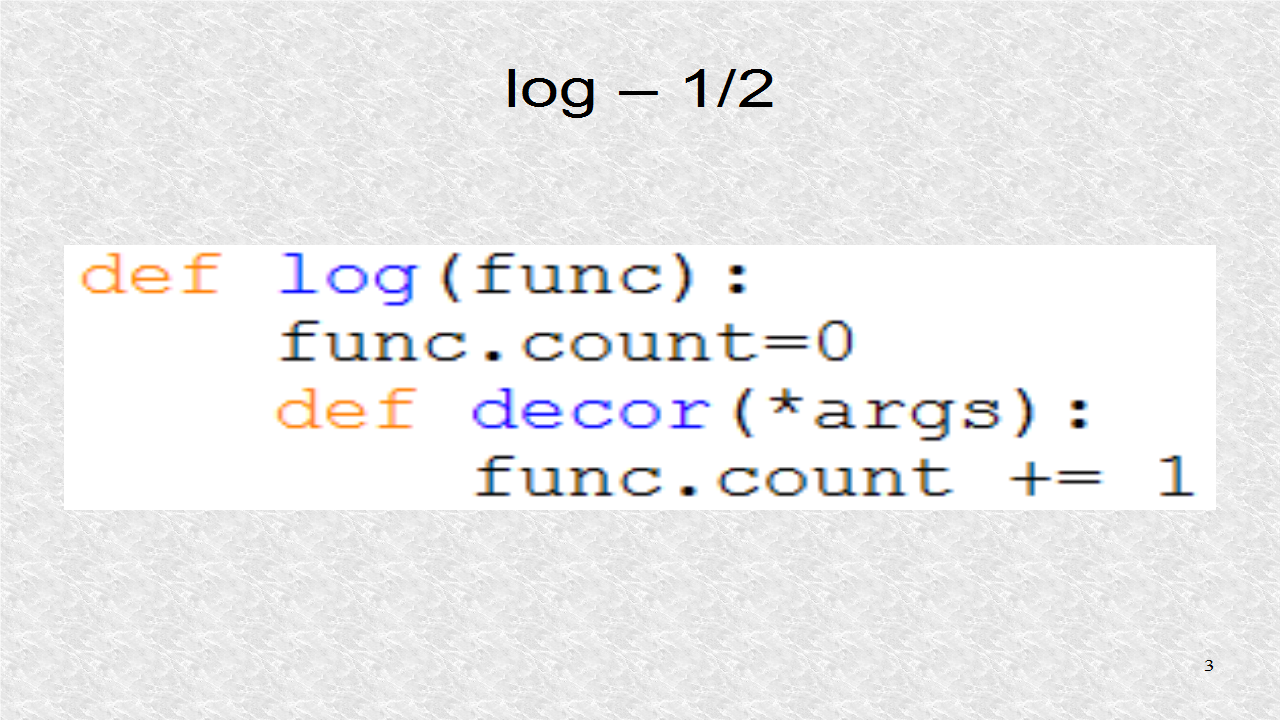
Next, it outputs some log statements. These logs, are sent to console, as well as a text file. The original function is executed in statement func(*args).
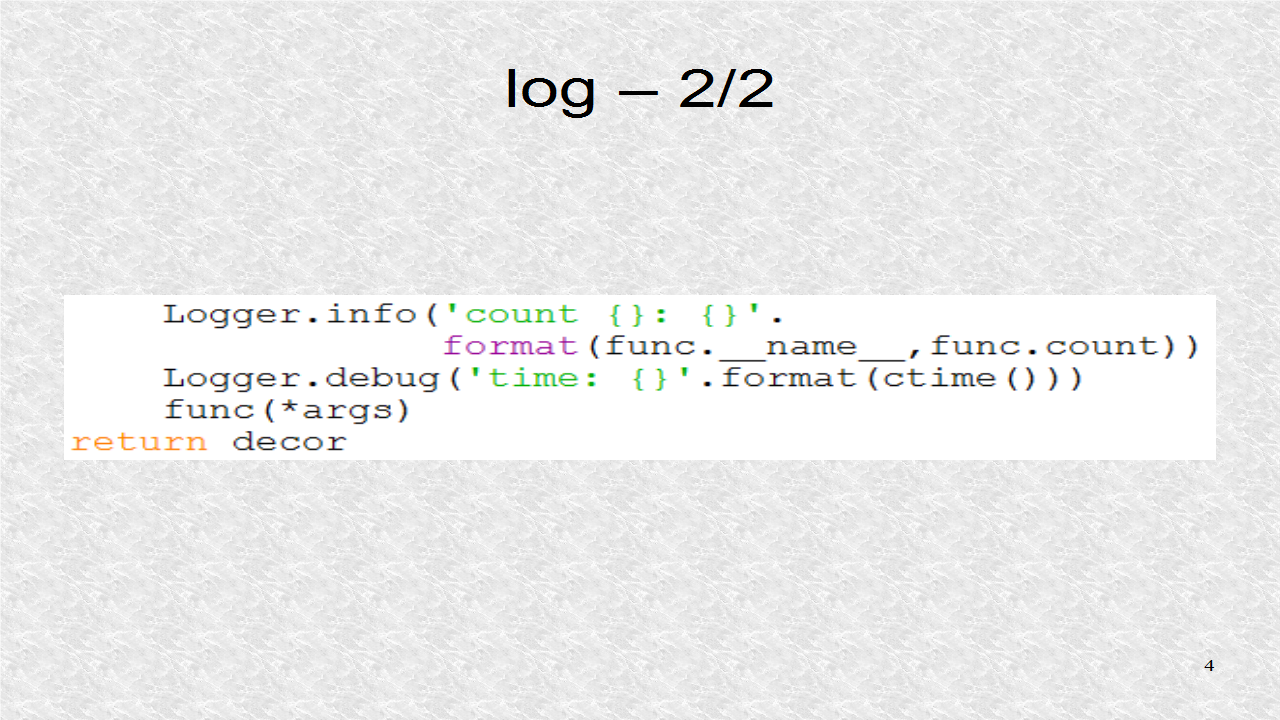
Inside the Ball widget class, the on_touch_down function, called by kivy whenever there is a click, is decorated by the log() function.
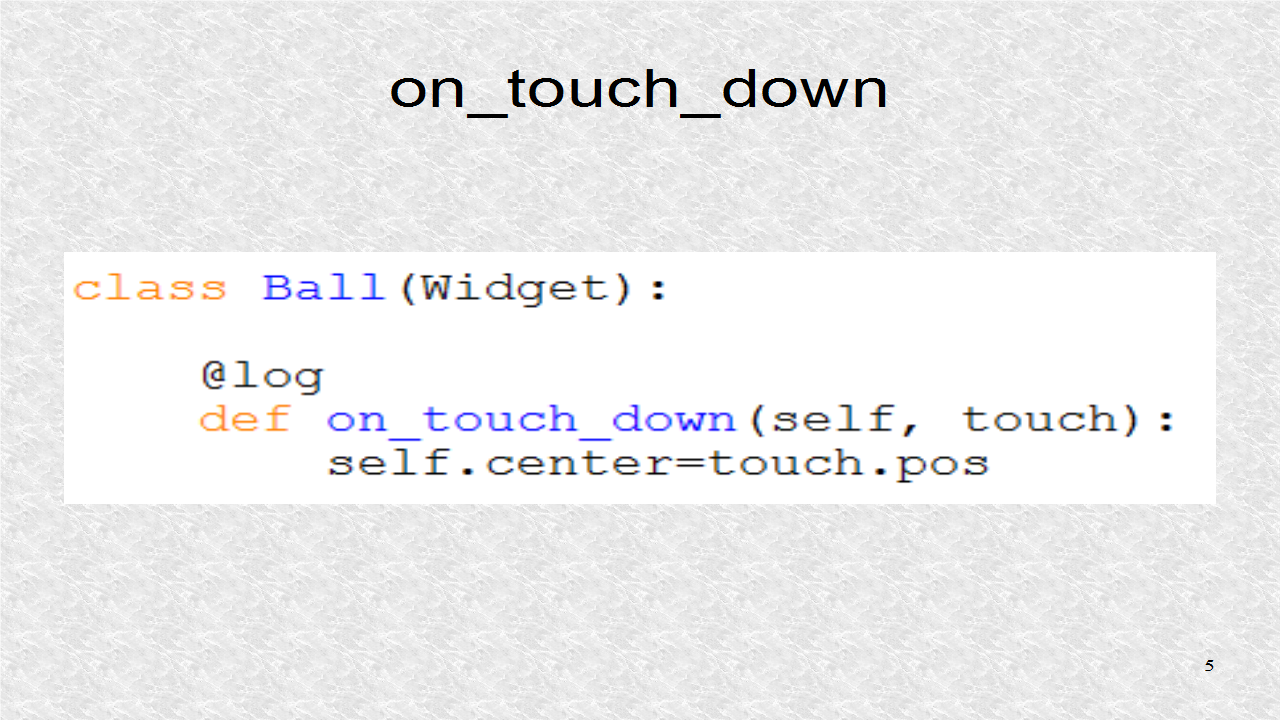
In addition, on_touch_move is also used. This will be called by kivy whenever there is dragging motion. There might be many such events in a short time duration. We will see log statements from these events.
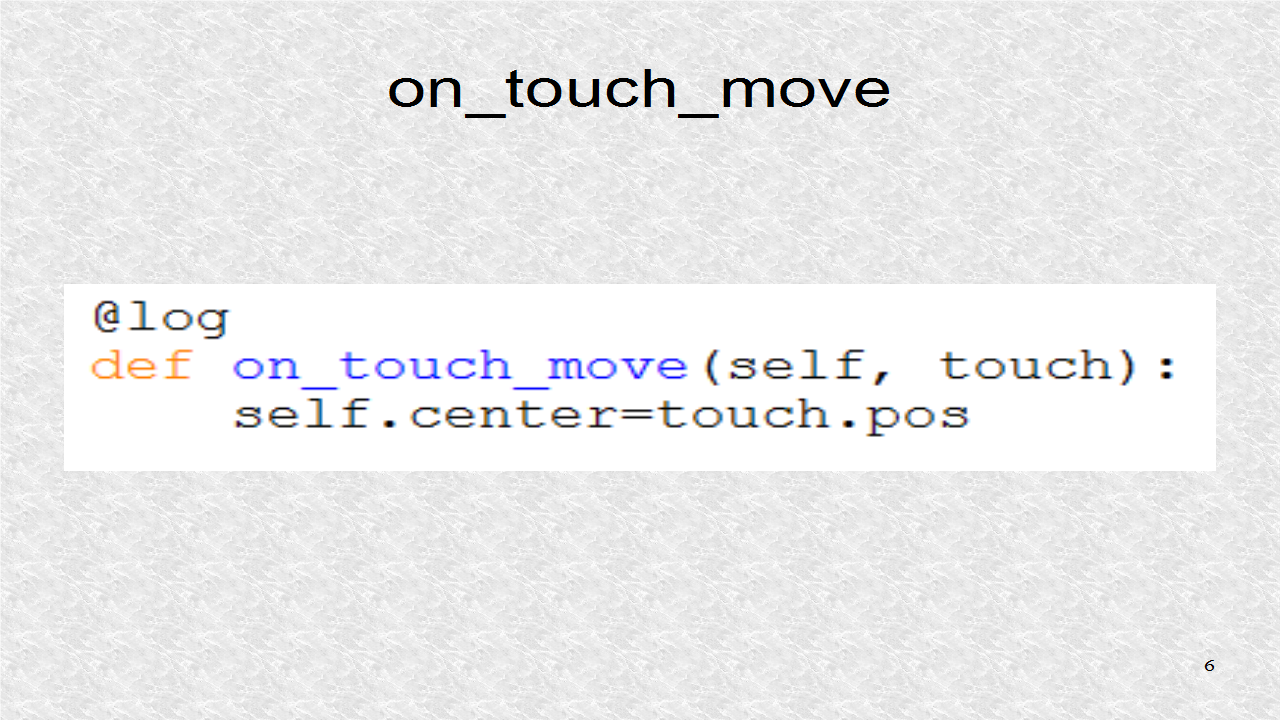
# ex52.py - Based on ex27.py from kivy.uix.widget import Widget from kivy.app import App from kivy.logger import Logger from time import ctime def log(func): func.count=0 def decor(*args): func.count += 1 Logger.info('count {}: {}'. format(func.__name__,func.count)) Logger.debug('time: {}'.format(ctime())) func(*args) return decor class Ball(Widget): @log def on_touch_down(self, touch): self.center=touch.pos @log def on_touch_move(self, touch): self.center=touch.pos class Ex52(Widget): pass class Ex52App(App): def build(self): return Ex52() if __name__=='__main__': Ex52App().run()
This is a partial log file output. The name of the log file is indicated near the top of console output. Usually the file, will be in the logs subfolder, of the .kivy folder, which will usually be hidden on Windows. You might want to search for logs, and then click to open that folder.
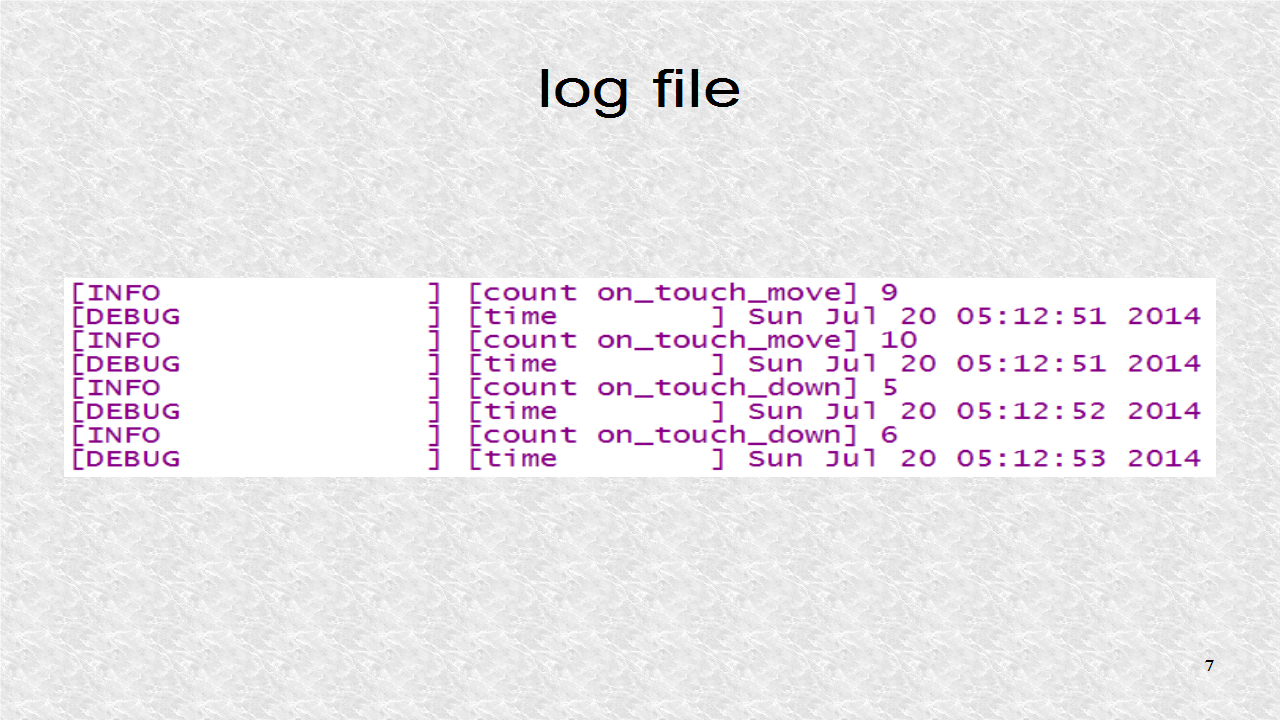
# ex52.kv <Ball>: size: 65,60 canvas: Color: rgb: .75, 0, 0 Ellipse: pos: self.pos size: self.size <Ex52>: canvas: Color: rgb: 0,0,1 Rectangle: size: root.width,root.height pos: 0,0 Label: pos: 300,root.top-100 text:'[size=32][color=8888CC]touch_down, touch_move[/color][/size]' markup: True Ball:
Python:
ReplyDeleteGood Post! Thank you so much for sharing this pretty post, it was so good to read and useful to improve my knowledge as updated one, keep blogging.
https://www.emexotechnologies.com/online-courses/python-training-in-electronic-city/
Good Post! Thank you so much for sharing this pretty post, it was so good to read and useful to improve my knowledge as updated one, keep blogging.
ReplyDeletePython Training in electronic city
nice information for beginners.thank you.
ReplyDeletejavacodegeeks
welookups python
I used to be more than happy to seek out this web-site.I wished to thanks for your time for this glorious learn!! I positively having fun with every little bit of it and I've you bookmarked to check out new stuff you blog post.mac reparieren berlin
ReplyDelete