If an application requires user data, we might display the FileChooser.
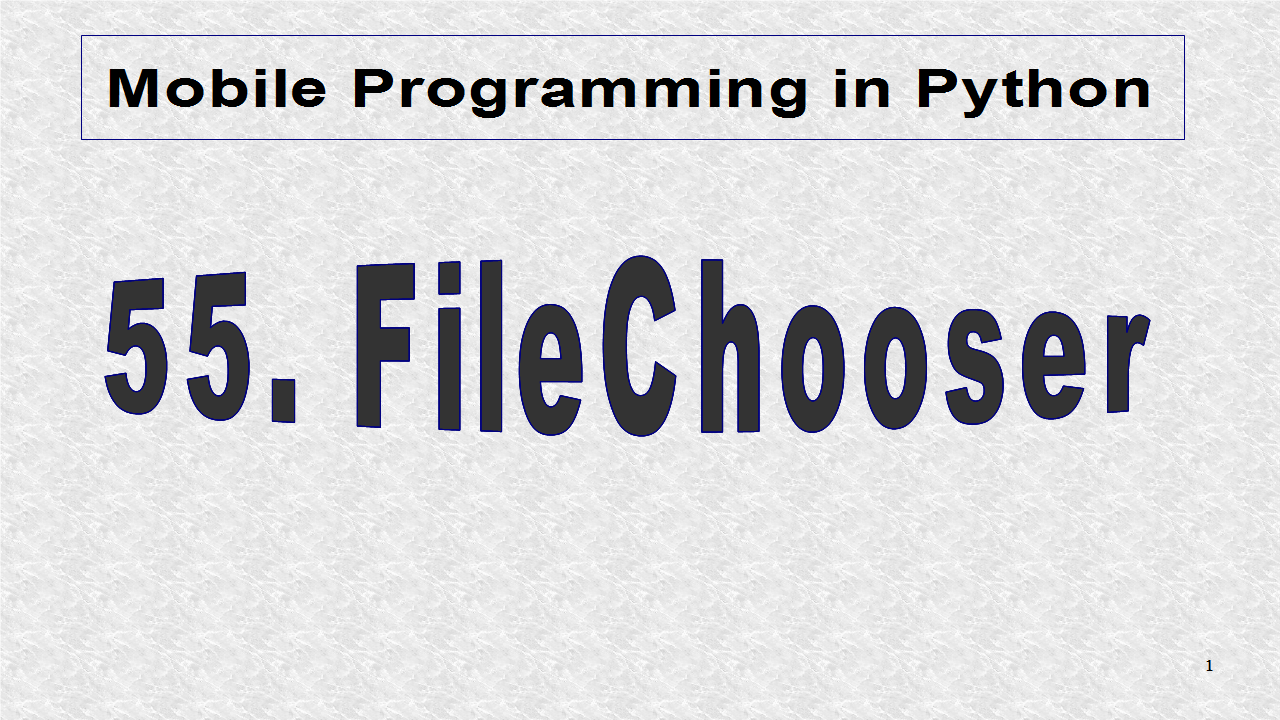
Since this is an application, we need the App class. The root is based on BoxLayout. The root has one function, select(). This will be called from the kv file, when the user selects any file. If a filename has been passed to select(), it will pass it to the label. label is defined in the kv file and is an attribute.
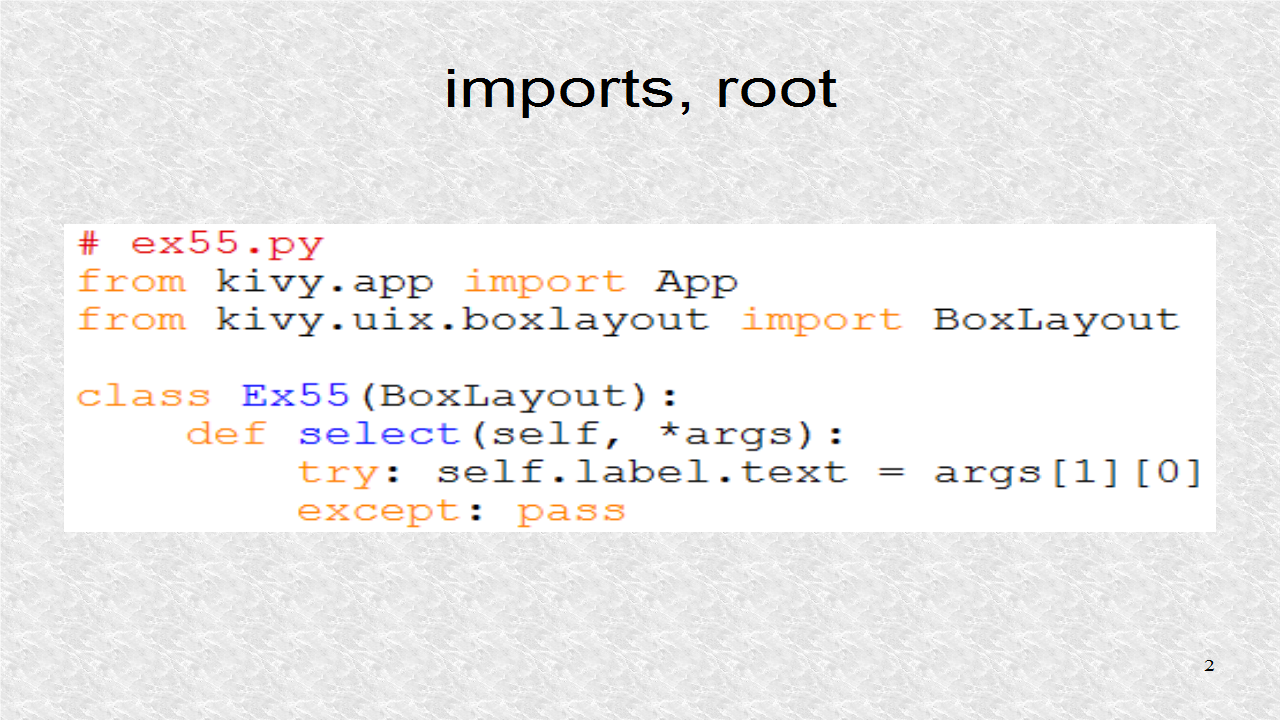
In the app class, we have to return the root class.
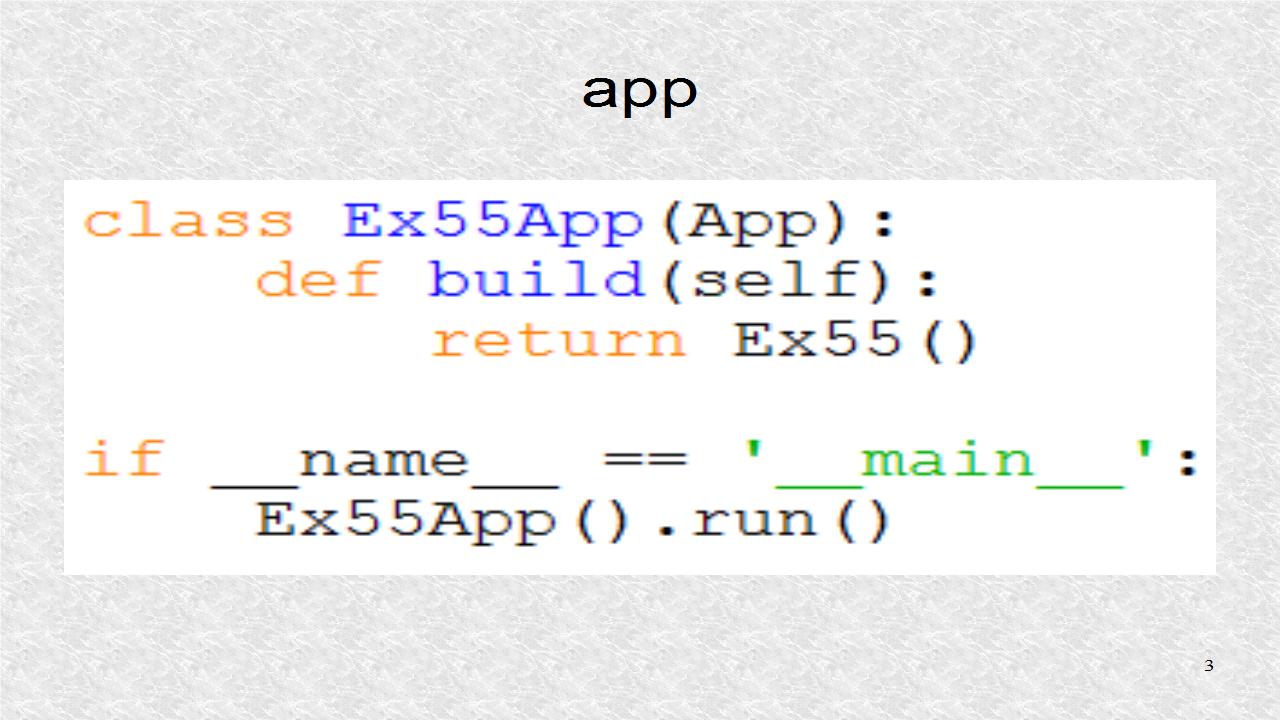
# ex55.py from kivy.app import App from kivy.uix.boxlayout import BoxLayout class Ex55(BoxLayout): def select(self, *args): try: self.label.text = args[1][0] except: pass class Ex55App(App): def build(self): return Ex55() if __name__ == '__main__': Ex55App().run()
In the kv file, we define the outer BoxLayout to have a 'vertical' orientation, and then we start the inner BoxLayout.
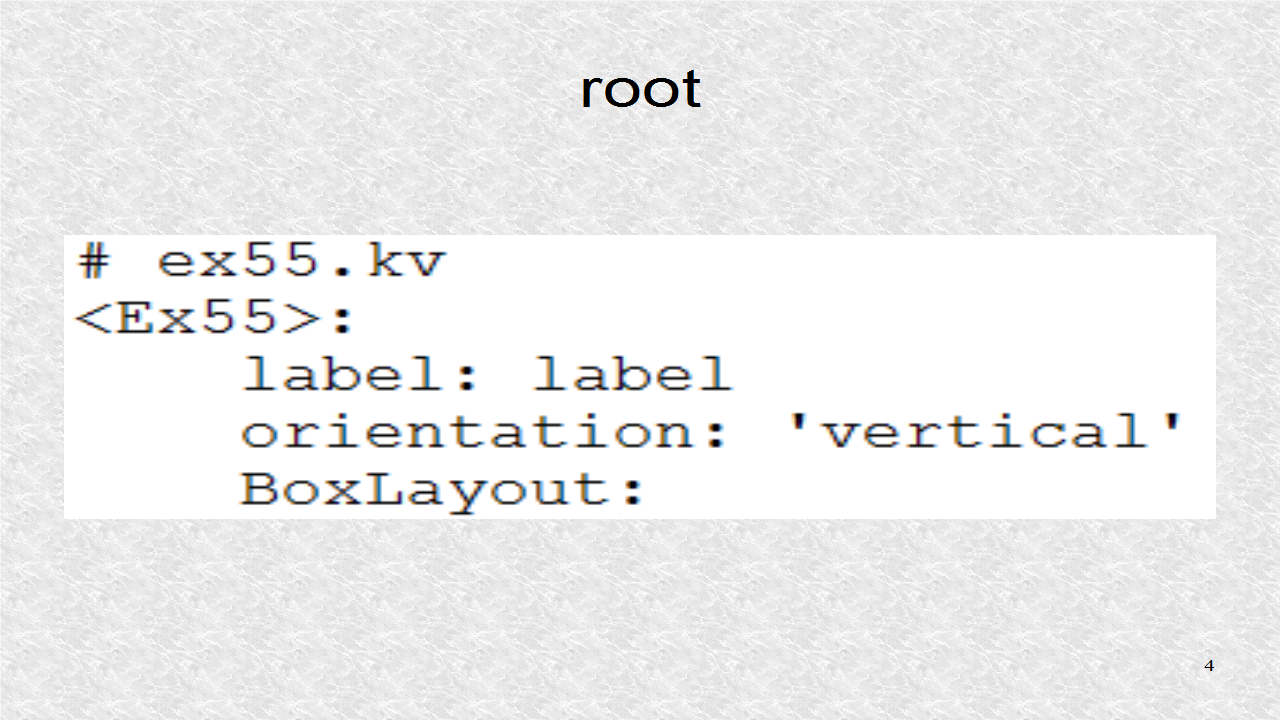
The inner BoxLayout has a default orientation of 'horizontal'. The first element is a FileChooserListView. We first set a background color. The on_selection is called, when the user selects a particular file, by clicking it. It will call the select() function.
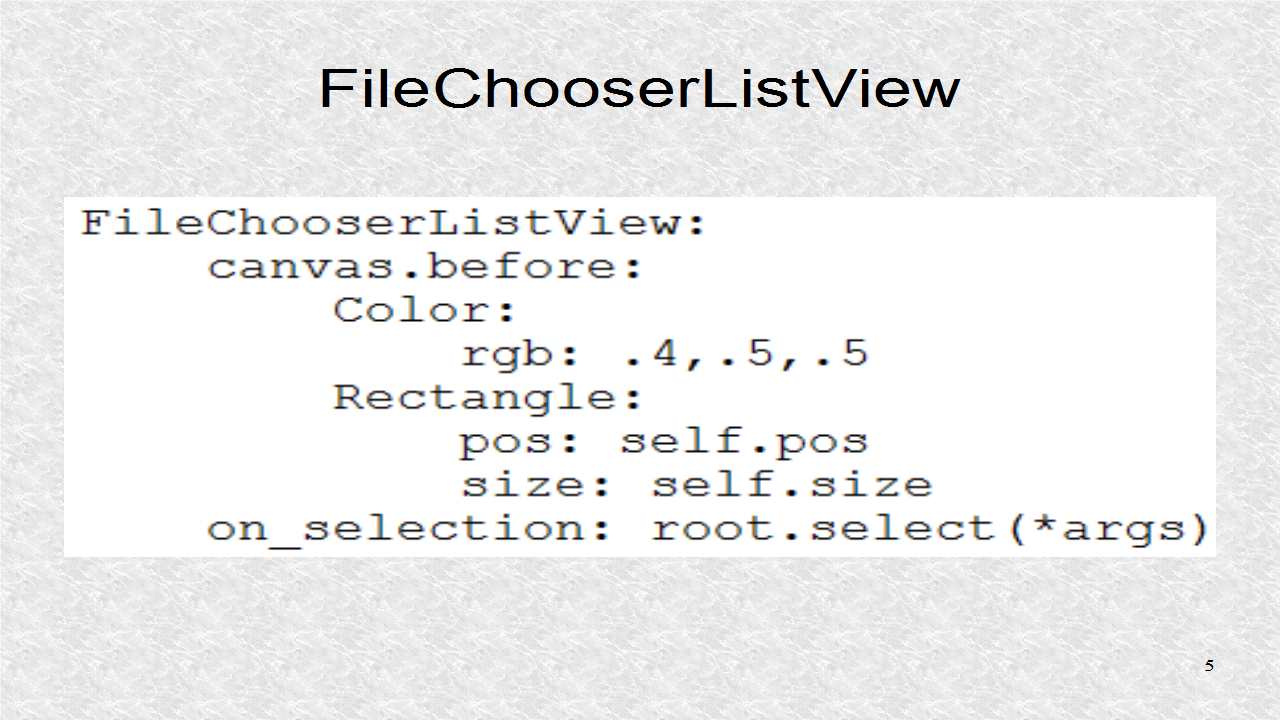
The next element in the Boxlayout is FileChooserIconView. We set a background color, and route the selected filename, to the select() function.
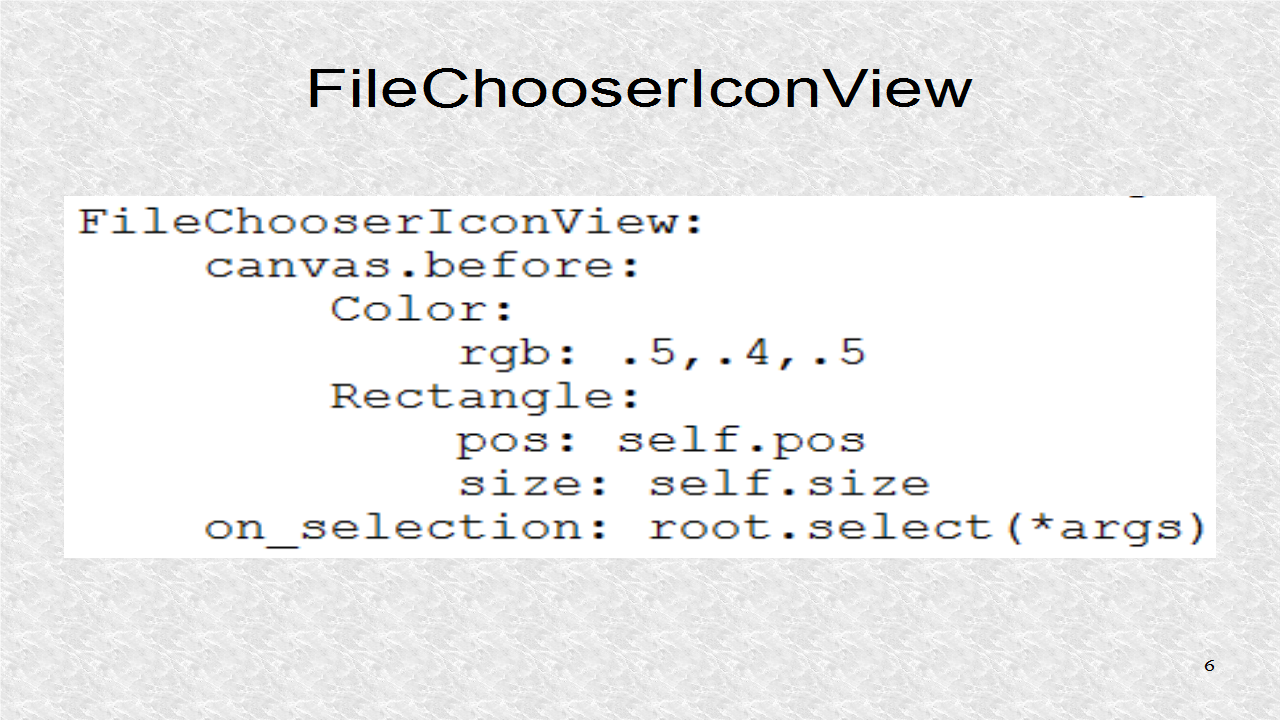
Finally, we have a Label. It has an ID of label, which was used earlier in the attribute definition. It has a small size_hint in y direction as it will occupy a small portion of the screen at the bottom. We then set a background color.
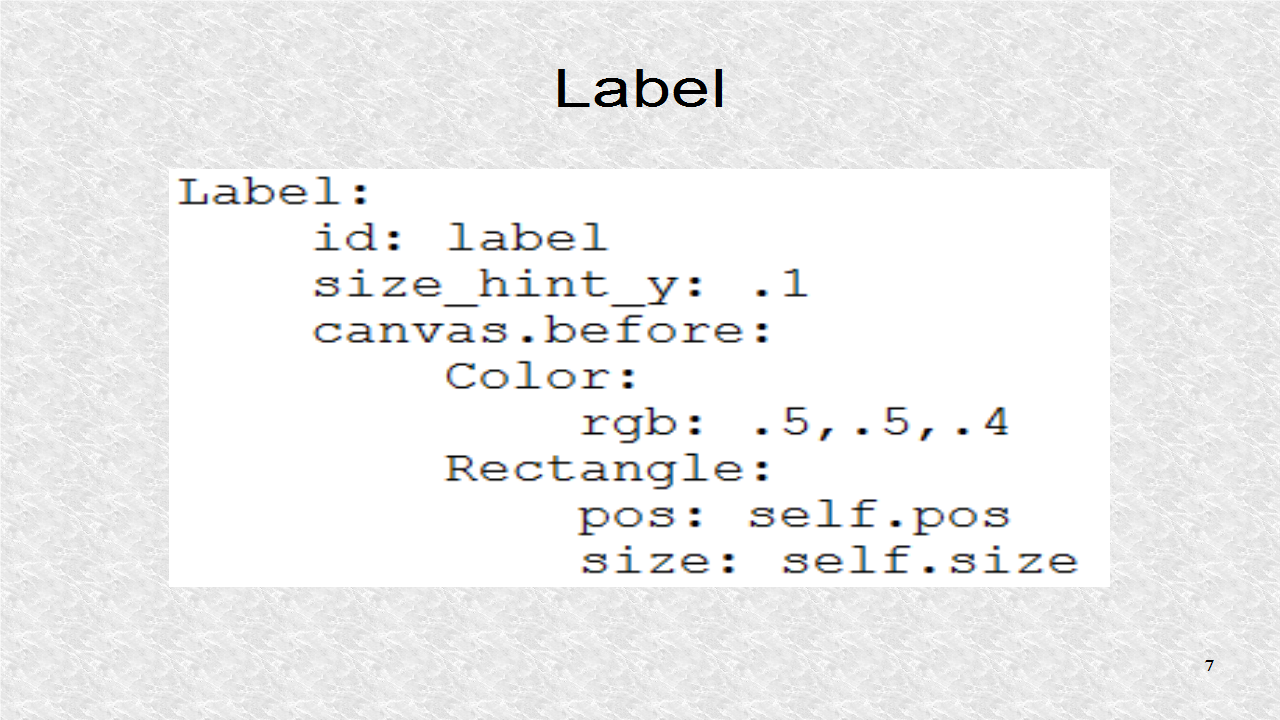
# ex55.kv <Ex55>: label: label orientation: 'vertical' BoxLayout: FileChooserListView: canvas.before: Color: rgb: .4,.5,.5 Rectangle: pos: self.pos size: self.size on_selection: root.select(*args) FileChooserIconView: canvas.before: Color: rgb: .5,.4,.5 Rectangle: pos: self.pos size: self.size on_selection: root.select(*args) Label: id: label size_hint_y: .1 canvas.before: Color: rgb: .5,.5,.4 Rectangle: pos: self.pos size: self.size
In the result, we can see the two FileChooser views, on the top-left, and top-right, which together, are about 90% of the screen area. These have different background colors, set in the kv file. The label on the bottom, indicates currently selected file.
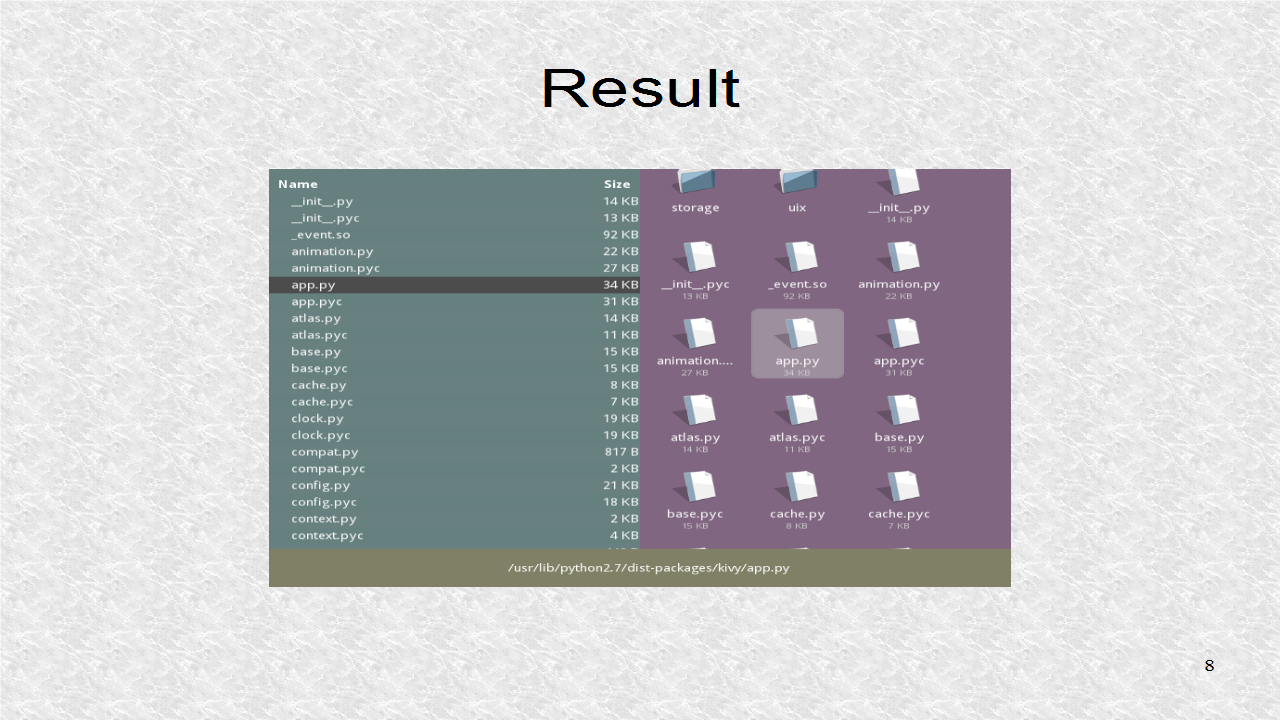
Good Post! Thank you so much for sharing this pretty post, it was so good to read and useful to improve my knowledge as updated one, keep blogging.
ReplyDeletePython Training in electronic city, Bangalore | #pythontraininginelectroniccity #pythontraining
DataSciencewithPythonTraininginelectroniccity,Bangalore | #datasciencewithpythontraininginelectroniccity #datasciencewithpythontraining
Python:
ReplyDeleteGood Post! Thank you so much for sharing this pretty post, it was so good to read and useful to improve my knowledge as updated one, keep blogging.
https://www.emexotechnologies.com/online-courses/python-training-in-electronic-city/
Good Post! Thank you so much for sharing this pretty post, it was so good to read and useful to improve my knowledge as updated one, keep blogging.
ReplyDeletePython Training in electronic city
nice article .thank you.
ReplyDeletefor more information visit
web programming tutorial
welookups
nice information for beginners.thank you.
ReplyDeletejavacodegeeks
welookups python
Well Explained. I am searching for the same. Thanks for sharing it here.
ReplyDeleteAlso , checkout my website if you want to know about computer guides and reviews then you can checkout my website clickhere
Sage 50 accounting software
ReplyDeleteHow to Reset Quickbooks Password?
Reset Quickbooks Password
Quickbooks Password change
Quickbooks won't open error
ReplyDeleteUICKBOOKS ERROR 3371 STATUS CODE 11118
Quickbooks online vs Quickbooks Desktop
Quickbooks error 9999
ReplyDeleteQuickbooks Error code 6000
Quickbooks Error 6000
Quickbooks Error 6000
Quickbooks Error code 9999
Today, while I was at work, my sister stole my iPad and tested to see if it can survive a twenty five foot drop, just so she can be a youtube sensation. My iPad is now broken and she has 83 views. I know this is entirely off topic but I had to share it with someone! apple service berlin
ReplyDelete